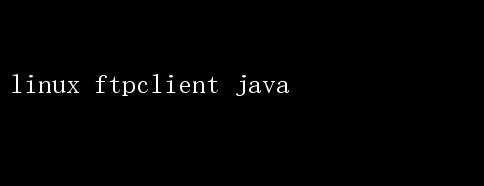
Linux FTP Client in Java: A Comprehensive Guide for Robust File Transfer
In the realm of network-based file transfer, the File Transfer Protocol(FTP) remains a cornerstone technology despite the advent of more modern alternatives. Its simplicity, widespread support, and robustness have ensured its enduring relevance, particularly in environments where interoperability and legacy systems are paramount. Java, with its cross-platform capabilities and extensive libraries, stands as an ideal programming language for developing FTP clients. This article delves into creating a robust FTP client in Java, emphasizing the intricacies of interaction with Linux-based FTP servers.
Introduction to FTP and Java
FTP is a standard network protocol used for the transfer of files over a TCP/IP-based network. It operates on two separate channels: a commandchannel (typically on port 21) for sending commands and receiving responses, and optionally, a data channel for file data transfer. Java provides multiple ways to interact with FTP servers, primarily through third-party libraries like Apache Commons Net, which simplifies the low-level socket programming required for FTP communication.
Why Use Java for FTP Client Development?
1.Cross-Platform Compatibility: Javas write once, run anywhere philosophy ensures that your FTP client will work seamlessly on any platform, including Windows, macOS, and Linux.
2.Extensive Libraries: Libraries like Apache Commons Net abstract away the complexities of FTP, allowing developers to focus on business logic.
3.Security: Javas robust security model can be leveraged to handle sensitive FTP credentials securely.
4.Scalability: Javas ability to handle large-scale applications makes it suitable for developing high-performance FTP clients.
Setting Up Your Development Environment
Before diving into code, ensure you have the necessary development environment set up:
1.JDK: Install the latest version of Java DevelopmentKit (JDK) from Oracle or AdoptOpenJDK.
2.IDE: Use an Integrated Development Environment(IDE) like IntelliJ IDEA, Eclipse, or NetBeans for ease of development.
3.Apache Commons Net: Add the Apache Commons Net library to your project. This can be done via Maven or by manually downloading the JAR file.
For Maven, include the following dependency inyour `pom.xml`:
commons-net
commons-net
3.8.0
Developing the FTP Client
Lets walk through the creation of a basic FTP client in Java using Apache Commons Net. This client will support basic functionalities such as connecting to an FTP server, logging in, listing files, uploading, and downloading files.
Step 1: Import Necessary Packages
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPReply;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
Step 2: Establishing a Connection
Creating an instance of`FTPClient` and establishing a connection involves setting the server address and port, and then logging in with the provided credentials.
public class FTPClientExample {
private FTPClient ftpClient = new FTPClient();
private String server = ftp.example.com;
private int port = 21;
private String user = yourUsername;
private String pass = yourPassword;
public void connect() throwsIOException {
ftpClient.connect(server,port);
boolean login = ftpClient.login(user, pass);
if(!FTPReply.isPositiveCompletion(ftpClient.getReplyCode())) {
ftpClient.disconnect();
throw new IOException(FTP server refusedconnection.);
}
if(!login) {
ftpClient.disconnect();
throw new IOException(Could not login to theserver.);
}
System.out.println(Connected to theserver);
}
public void disconnect() throwsIOException {
if(ftpClient.isConnected()){
ftpClient.logout();
ftpClient.disconnect();
}
}
}
Step 3: Listing Files
To list files in a specific directory on the FTP server, use the`listFiles` method.
public void listFiles(String remoteDir) throwsIOException {
ftpClient.changeWorkingDirectory(remoteDir);
FTPFile【】 files = ftpClient.listFiles();
if(files!= null && files.length > {
for(FTPFile file : files) {
String details =(file.isDirectory() ? D :-) + + file.getName();
System.out.println(details);
}
}else {
System.out.println(No files found in thedirectory.);
}
}
Step 4: Uploading Files
Uploading a file involves creating an input stream from the local file and usingthe `storeFile` method.
public void uploadFile(String remoteFilePath, String localFilePath) throwsIOException {
try(InputStream inputStream = new FileInputStream(localFilePath)){
boolean done = ftpClient.storeFile(remoteFilePath, inputStream);
if(done) {
System.out.println(The file is uploaded successfully.);
}else {
System.out.println(Could not upload the file.);
}
}
}
Step 5: Downloading Files
Downloading a file is similar to uploading but uses an output stream and the`retrieveFile` method.
public void downloadFile(String remoteFilePath, String localFilePath) throwsIOException {
try(OutputStream outputStream = newFileOutputStream(localFilePath)) {
boolean success = ftpClient.retrieveFile(remoteFilePath, outputStream);
if(success) {
System.out.println(The file is downloaded successfully.);
}else {
System.out.println(Could not download the file.);
}
}
}
Step 6: Complete FTP Client Example
Combining all the methods into a complete FTP client class allows for com