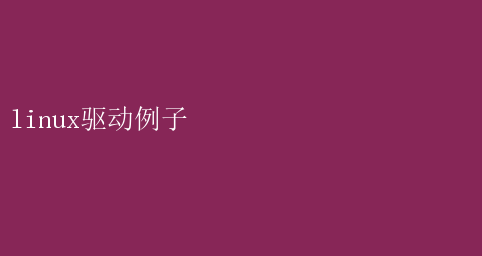
Linux驱动开发:从理论到实践的深度探索——以一个实际例子为线索
在当今的信息技术领域中,Linux操作系统凭借其开源、稳定、高效的特点,在服务器、嵌入式系统、云计算等多个领域占据了举足轻重的地位
而Linux驱动作为操作系统与硬件设备之间的桥梁,其重要性不言而喻
本文将通过一个具体的Linux驱动开发例子,深入浅出地探讨Linux驱动开发的全过程,旨在帮助读者理解Linux驱动开发的原理、流程与技巧,为成为一名优秀的Linux驱动开发者打下坚实基础
一、Linux驱动开发基础
1.1 Linux驱动概述
Linux驱动,简而言之,就是一组用于控制硬件设备、使其能够被Linux操作系统正确识别并使用的软件代码
Linux驱动分为字符设备驱动、块设备驱动和网络设备驱动三大类,每一类都有其特定的应用场景和编程接口
1.2 驱动加载与卸载
在Linux系统中,驱动的加载与卸载通常通过`insmod`(或`modprobe`)和`rmmod`命令实现
加载驱动时,系统会调用驱动的`init`或`probe`函数进行初始化;卸载时,则调用`exit`或`remove`函数进行资源释放
1.3 内核模块机制
Linux驱动通常以内核模块的形式存在,这使得驱动可以在不重启系统的情况下被动态加载和卸载,极大提高了系统的灵活性和可维护性
二、实战:LED驱动开发
为了更直观地展示Linux驱动开发过程,我们将以一个简单的LED驱动为例,详细讲解从需求分析到代码实现的全过程
2.1 需求分析
假设我们有一块开发板,板上有一个LED灯,我们需要通过Linux驱动来控制这个LED灯的亮灭
2.2 硬件接口
首先,我们需要了解LED灯连接在开发板的哪个GPIO(通用输入输出)引脚上
假设LED连接在GPIO17上
2.3 驱动设计
- 设备文件:为LED创建一个设备文件,如`/dev/led`,用户空间程序通过该文件控制LED
- 控制接口:提供简单的读写接口,写入1点亮LED,写入0熄灭LED
- 资源管理:在驱动加载时申请GPIO资源,卸载时释放
2.4 代码实现
2.4.1 头文件与宏定义
include
include
include
include
include
include
include
defineDEVICE_NAME led
defineCLASS_NAME leds
defineLED_GPIO 17
2.4.2 驱动入口与出口
static int__initled_init(void){
printk(KERN_INFO LED Driver Initializingn);
// 初始化代码
return 0; // 成功
}
static void__exitled_exit(void){
printk(KERN_INFO LED Driver Exitingn);
// 清理代码
}
module_init(led_init);
module_exit(led_exit);
MODULE_LICENSE(GPL);
MODULE_AUTHOR(Your Name);
MODULE_DESCRIPTION(A Simple LED Driver for Linux);
MODULE_VERSION(1.0);
2.4.3 GPIO初始化与释放
static int major;
static structclass led_class;
static structdevice led_device;
static intled_gpio;
static intled_probe(struct platform_devicepdev) {
led_gpio = of_get_named_gpio(pdev->dev.of_node, gpio-led, 0);
if(led_gpio < {
printk(KERN_ERR Failed to get LED GPIO
);
return -ENODEV;
}
if(gpio_request(led_gpio, LED) < {
printk(KERN_ERR Failed to request LED GPIO
);
return -ENODEV;
}
gpio_direction_output(led_gpio, 0); // 初始状态熄灭
major = register_chrdev(0, DEVICE_NAME, &led_fops);
if(major < {
printk(KERN_ERR Failed to register character device
);
gpio_free(led_gpio);
return major;
}
led_class = class_create(THIS_MODULE, CLASS_NAME);
if(IS_ERR(led_class)) {
unregister_chrdev(major, DEVICE_NAME);
gpio_free(led_gpio);
returnPTR_ERR(led_class);
}
led_device = device_create(led_class, NULL, MKDEV(major, 0), NULL, DEVICE_NAME);
if(IS_ERR(led_device)) {
class_destroy(led_class);
unregister_chrdev(major, DEVICE_NAME);
gpio_free(led_gpio);
returnPTR_ERR(led_device);
}
printk(KERN_INFO LED Driver Probed Successfully
);
return 0;
}
static intled_remove(struct platform_devicepdev) {
device_destroy(led_class, MKDEV(major, 0));
class_unregister(led_class);
class_destroy(led_class);
unregister_chrdev(major, DEVICE_NAME);
gpio_free(led_gpio);
printk(KERN_INFO LED Driver Removed
);
return 0;
}
2.4.4 文件操作接口
static ssize_tled_write(struct filefile, const char __user buf,size_t len, loff_toffset) {
char command;
if(copy_from_user(&command, buf, 1)){
return -EFAULT;
}
if(command == 1){
gpio_set_value(led_gpio, 1); // 点亮LED
} else if(command == 0){
gpio_set_value(led_gpio, 0); // 熄灭LED
}else {
printk(KERN_WARNING Invalid commandn);
return -EINVAL;
}
return len;
}
static const struct file_operationsled_fops ={
.owner =THIS_MODULE,
.write =led_write,
};
2.4.5 设备树配置
在设备树文件(.dts)中添加LED的GPIO配置:
leds {
compatible = your,led;
gpio-led = <&gpio1 17GPIO_ACTIVE_HIGH>;
};