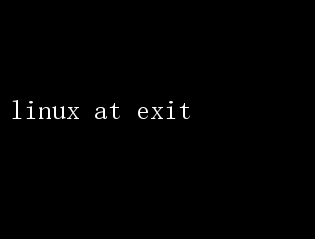
Linux at Exit: Ensuring Graceful Termination of Processes and Systems
In the realm of computing, the art of handling exits gracefully is paramount to maintaining system stability, data integrity, and user satisfaction. Linux, as a robust and versatile operating system, offers a sophisticated suite of mechanisms to manage process termination and system shutdown. Understanding how Linux handles at exit scenarios is crucial for both system administrators and developers tasked with ensuring that applications and systems behave predictably and efficiently. This article delves into the intricacies of Linux at exit, exploring the processes, tools, and best practices that underpin graceful termination.
The Importance of Graceful Termination
Graceful termination refers to the orderly shutdown of a process or system, ensuring that all necessary cleanup operations are performed, resources are released, and data is saved before the process or system ceases operation. This is contrasted with abrupt termination, which can lead to resource leaks, data corruption, and potential system instability.
In Linux, graceful termination is particularly significant due to its multi-user, multi-tasking nature. With numerous processes running concurrently, each responsible for various tasks, ensuring that they exit cleanly is vital for overall system health. Whether its a user-initiated process termination, a system shutdown due to maintenance, or an unexpected power loss, Linux provides mechanisms to handle these scenarios seamlessly.
Signals: The Backbone of Process Termination
At the heart of Linuxs process termination mechanism lies the signal system. Signals are software interrupts that allow the operating system or one process to notify another process of an event or request. When it comes to termination, the most relevant signals are:
1.SIGTERM (15): The default signal sent to a process to request its termination. Processes can catch this signal to perform any necessary cleanup before exiting.
2.SIGKILL (9): This signal cannot be caught, blocked, or ignored. It immediately terminates the process. SIGKILL should be used only as a last resort when SIGTERM fails to terminate a process.
3.SIGINT (2): Typically sent when a user interrupts a process, such as by pressing Ctrl+C in a terminal. Like SIGTERM, it can be caught and handled by the process.
When a process receives a termination signal, it can either ignoreit (not recommended for SIGTERM or SIGKILL), handle it by executing a custom cleanup routine, or default to the operating systems termination behavior.
Handling Signals in Programs
Developers can program their applications to handle termination signals gracefully by using signal handling functions provided by the C standard library or similar mechanisms in other programming languages. For instance, in C, the`signal()` or`sigaction()` functions can be used to register a handler for SIGTERM or SIGINT.
include
include
include
include
void handle_term_signal(intsig){
printf(Received signal %d, performing cleanup...
, sig);
// Perform necessary cleanup actions
// ...
exit(0);// Exit gracefully after cleanup
}
int main() {
// Register signal handler for SIGTERM and SIGINT
signal(SIGTERM, handle_term_signal);
signal(SIGINT, handle_term_signal);
// Simulate a long-running process
while(1) {
printf(Process running...n);
sleep(1);
}
return 0;
}
In this example,the `handle_term_signal` function is called when the process receives SIGTERM or SIGINT, allowing it to perform any necessary cleanup before exiting.
System Shutdown:The `shutdown` Command
On the system level, Linux provides the`shutdown` command for initiating a controlled shutdown. This command sends appropriate signals to all processes, giving them a chance to terminate gracefully before the system powers down.
The `shutdown` command can be configured to:
- Schedule a shutdown at a specific time.
- Initiate an immediate shutdown.
- Specify a shutdown message that will be broadcast to all logged-in users.
- Use different runlevels ortargets (e.g., reboot, halt, power-off) to define the final state of the system.
For example, to initiate an immediate shutdown and power off the system, you would use:
sudo shutdown -h now
Conversely, to schedule a shutdown for 10 minutes later with a custom message:
sudo shutdown -h +10 System maintenance scheduled. Please save your work.
During the shutdown process, `shutdown` sends warningsignals (e.g., SIGTERM) to all running processes, allowing them to terminate gracefully. If processes do not respond within a specified timeframe,`shutdown` escalates to SIGKILL to force termination.
`systemd` and Service Management
Modern Linux distributions oftenuse `systemd` as their system and service manager. `systemd` introduces additional layers of control over service lifecycle management, including handling service exits.
When a`systemd` service is stopped or restarted, `systemd` sends the appropriate signals to the services main process. By default, `systemd` sends SIGTERM, followed by SIGKILL if the service does not terminate within the`TimeoutStopSec` timeout. Services can define custom handlers and timeouts in their unit files to better control their termination behavior.
For example, a`systemd` service unit file might look like this:
【Unit】
Description=My Custom Service
【Service】
ExecStart=/usr/bin/my_service_daemon
ExecStop=/usr/bin/my_service_stop_script
TimeoutStopSec=30
【Install】
WantedBy=multi-user.target
In this unit file, `ExecStop` specifies a custom stop script that can be used to perform additional cleanup actions.`TimeoutStopSec` defines the timeoutbefore `systemd` escalates to SIGKILL.
Logging and Monitoring
Ensuring graceful termination also involves logging and monitoring. By logging termination events, system administrators can troubleshoot issues that prevent processes from exiting cleanly. Logging can be achi