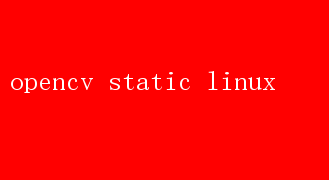
OpenCV Static Libraries on Linux: A Comprehensive Guide for Developers
In the vast landscape of computer vision and image processing libraries, OpenCV stands as a towering giant. With its rich feature set, robust algorithms, and extensive documentation, OpenCV has become the go-to choice for developers across various domains. Whether youre working on facial recognition, autonomous driving, or real-time video processing, OpenCV has the tools you need.
One of the key decisions youll face when integrating OpenCV into your Linux-based project is whether to use the static or dynamic libraries. While dynamiclibraries (shared libraries) offer certain advantages in terms of memory usage and updates, static libraries(statically linkedlibraries) provide a level of control and stability that can be invaluable in certain scenarios. In this article, well delve into the intricacies of using OpenCV static libraries on Linux, highlighting their benefits, installation steps, and tips for efficient use.
The Advantages of Static Libraries
Before we dive into the specifics of setting up OpenCV static libraries on Linux, lets understand why you might prefer them over dynamic libraries.
1.Dependency Management: Static linking embeds all necessary code directly into your executable. This eliminates the need for external dependencies, making your application more portable and easier to distribute. You dont have to worry about library versions or compatibility issues on target machines.
2.Performance: While the performance difference between static and dynamic libraries is often negligible, static linking can sometimes result in slightly faster execution due to reduced overhead from dynamic linking and potential elimination of certain runtime checks.
3.Security: Static linking can improve security by reducing the attack surface. Since all code is bundled within the executable, theres no risk of an attacker exploiting vulnerabilities in shared libraries that your application depends on.
4.Consistency: Static linking ensures that the version of the library your application uses is the one you tested against. This can prevent subtle bugs caused by unexpected changes in library behavior across versions.
Setting Up OpenCV Static Libraries on Linux
Now, lets walk through the process of installing and configuring OpenCV static libraries on a Linux system.
Step 1: Install Dependencies
Before compiling OpenCV from source, you need to install some essential dependencies. These include build tools, libraries, and headers required for compilation.
sudo apt-get update
sudo apt-get install build-essential cmake git libgtk2.0-dev pkg-config libavcodec-dev libavformat-dev libswscale-dev
sudo apt-get install python3-dev python3-numpy libtbb2 libtbb-dev libjpeg-dev libpng-dev libtiff-dev
sudo apt-get install libdc1394-22-dev
Step 2: Download OpenCV Source Code
Next, clone the OpenCV repository from GitHub. This will give you the latest development version. If you need a specific release, you can download a tarball from the OpenCV website instead.
cd ~
git clone https://github.com/opencv/opencv.git
cd opencv
git checkout
Optional, if you want a specific version
Additionally, if you plan to use OpenCVs contributed modules(which include extra algorithms andfeatures), you should also clone the opencv_contrib repository:
cd ~
git clone https://github.com/opencv/opencv_contrib.git
Step 3: Configure CMake for Static Libraries
Create a build directory and run CMake to configure the build process. Specify that you want to build static libraries by setting the`BUILD_SHARED_LIBS` optionto `OFF`.
cd opencv
mkdir build
cd build
cmake -D CMAKE_BUILD_TYPE=Release
-D CMAKE_INSTALL_PREFIX=/usr/local
-D OPENCV_EXTRA_MODULES_PATH=~/opencv_contrib/modules
-D BUILD_SHARED_LIBS=OFF
-D WITH_GTK=ON
-D WITH_TBB=ON
-D WITH_V4L=ON
-D WITH_OPENMP=ON
-D WITH_FFMPEG=ON
-D WITH_DC1394=ON
..
Adjust the`WITH_` options as needed to include or exclude additional features based on your requirements.
Step 4: Compile and Install
With CMake configured, you can now compile OpenCV. This step can take some time, depending on your systems performance.
make -j$(nproc)
sudo make install
The `-j$(nproc)` optiontells `make` to use all available CPU cores for compilation, speeding up the process.
Step 5: Verify Installation
To ensure that OpenCV static libraries have been installed correctly, you can check the installation directory. The static libraries should have a`.a` extension.
ls /usr/local/lib/ | grep opencv
You should see fileslike `libopencv_core.a,libopencv_imgproc.a`, and so on.
Integrating OpenCV Static Libraries into Your Project
Now that OpenCV static libraries are installed, you need to integrate them into your project. This involves configuring your build system to link against the static libraries instead of the shared ones.
Example with CMake
If youre using CMake for your project, you can specify the OpenCV static libraries like this:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
find_package(Required OpenCVREQUIRED)
include_directories(${OpenCV_INCLUDE_DIRS})
add_executable(MyExecutable main.cpp)
target_link_libraries(MyExecutable
${OpenCV_LIBS}This will link against the static libraries if BUILD_SHARED_LIBS=OFF was used during OpenCV compilation
)
Make sure to adjustthe `OpenCV_LIBS` variable if you only need specific OpenCV modules.
Example with Makefile
If youre using a traditional Makefile, youll need to manually specify the paths to the static libraries and include directories:
CXX = g++
CXXFLAGS = -std=c++11 -I/usr/local/include/opencv4
LDFLAGS = -L/usr/local/lib -lopencv_core -lopencv_imgproc -lopencv_highgui -lopencv_imgcodecsAdd other modules as needed
SRC = main.cpp
OBJ =$(SRC:.cpp=.o)
all: MyExecutable
MyExecutable: $(OBJ)
$(CXX)$(OBJ) -o $@$(LDFLAGS)
.cpp.o:
$(CXX)$(CXXFLAGS) -c $< -o $@
clean:
rm -f$(OBJ) MyExecutable
Tips for Efficient Use of OpenCV Static Libraries
1.Minimize Dependencies: Only link against the OpenCV modules you actually need. This will reduce the size of your executable and improve load times.
2.Optimize Compilation: Use compileroptimizations (e.g.,`-O2`,`-O3`) to improve the performance of your application. Rem